728x90
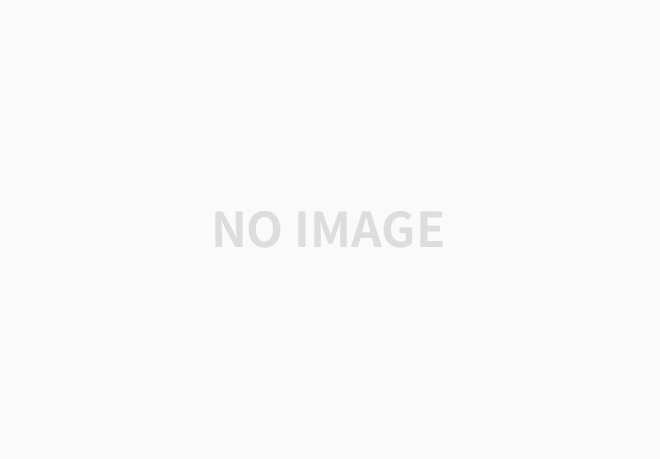
문제
Given the root node of a binary search tree (BST) and a value. You need to find the node in the BST that the node's value equals the given value. Return the subtree rooted with that node. If such node doesn't exist, you should return NULL.
For example,
Given the tree:
4
/ \
2 7
/ \
1 3
And the value to search: 2
You should return this subtree:
2
/ \
1 3
In the example above, if we want to search the value 5, since there is no node with value 5, we should return NULL.
Note that an empty tree is represented by NULL, therefore you would see the expected output (serialized tree format) as [], not null.
BST 의 특성
root node 기준으로 작은건 왼쪽 큰건 오른쪽인 특성을 이용한다.
Iteratively
/**
* Definition for a binary tree node.
* public class TreeNode {
* public var val: Int
* public var left: TreeNode?
* public var right: TreeNode?
* public init() { self.val = 0; self.left = nil; self.right = nil; }
* public init(_ val: Int) { self.val = val; self.left = nil; self.right = nil; }
* public init(_ val: Int, _ left: TreeNode?, _ right: TreeNode?) {
* self.val = val
* self.left = left
* self.right = right
* }
* }
*/
class Solution {
func searchBST(_ root: TreeNode?, _ val: Int) -> TreeNode? {
if root == nil {
return nil
}
var subRoot : TreeNode? = root
while (subRoot != nil){
if subRoot!.val == val {
return subRoot
}
if subRoot!.val > val {
subRoot = subRoot!.left
}else {
subRoot = subRoot!.right
}
}
return nil
}
}
Recursive Way
/**
* Definition for a binary tree node.
* public class TreeNode {
* public var val: Int
* public var left: TreeNode?
* public var right: TreeNode?
* public init() { self.val = 0; self.left = nil; self.right = nil; }
* public init(_ val: Int) { self.val = val; self.left = nil; self.right = nil; }
* public init(_ val: Int, _ left: TreeNode?, _ right: TreeNode?) {
* self.val = val
* self.left = left
* self.right = right
* }
* }
*/
class Solution {
func searchBST(_ root: TreeNode?, _ val: Int) -> TreeNode? {
guard let root = root else {
return nil
}
if root.val == val {
return root
}
if val < root.val {
return searchBST(root.left , val)
}else {
return searchBST(root.right, val)
}
}
}
728x90
'Algorithm > LeetCode' 카테고리의 다른 글
LeetCode - Fibonacci Number, Climbing Stairs (0) | 2020.09.10 |
---|---|
LeetCode - Pascal's Triangle II (0) | 2020.09.10 |
LeetCode - Reverse Linked List 🤦🏻 (0) | 2020.09.09 |
LeetCode - Swap Nodes in Pairs (0) | 2020.09.03 |
LeetCode - Reverse String (0) | 2020.09.03 |
댓글