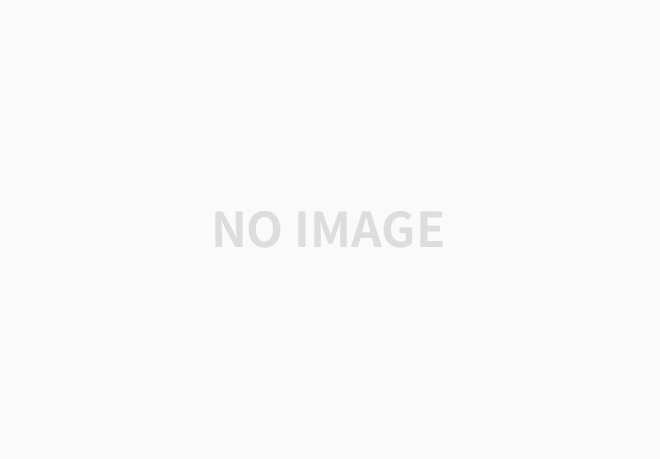
How to effectively use LeetCode to prepare for interviews!! - LeetCode Discuss
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
https://leetcode.com/problems/roman-to-integer/
Roman to Integer - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제
Roman numerals are represented by seven different symbols: I, V, X, L, C, D and M.Symbol Value I 1 V 5 X 10 L 50 C 100 D 500 M 1000
For example, two is written as II in Roman numeral, just two one's added together. Twelve is written as, XII, which is simply X + II. The number twenty seven is written as XXVII, which is XX + V + II.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not IIII. Instead, the number four is written as IV. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as IX. There are six instances where subtraction is used:
- I can be placed before V (5) and X (10) to make 4 and 9.
- X can be placed before L (50) and C (100) to make 40 and 90.
- C can be placed before D (500) and M (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer. Input is guaranteed to be within the range from 1 to 3999.
Example 1:
Input: "III" Output: 3
Example 2:
Input: "IV" Output: 4
Example 3:
Input: "IX" Output: 9
Example 4:
Input: "LVIII" Output: 58 Explanation: L = 50, V= 5, III = 3.
Example 5:
Input: "MCMXCIV" Output: 1994 Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
로마자를 정수로바꾸는 문제다.
I X C의 심볼은 뒤에 특정 로마자가 오는 것 을 제외하면 정해진 값을 더해주기만 하면되고 특정 로마자는 6가지 경우로 따로 처리해 주면 된다. 생각하면서 조금 힘들었던 건 charAt(i) 와 charAt(i+1)의 부분이 s.length()-1 때는 i+1이 index 에러가 나는 것이였다. 정말 똑같은 코드를 두번 쓰기 싫었지만 마지막 index를 처리하기 위해서 switch문을 두번 사용하였다.... 부끄럽다..
class Solution {
public int romanToInt(String s) {
int answer = 0;
for (int i = 0; i < s.length(); i++) {
if (i == s.length() - 1) {
System.out.println(s.charAt(i));
switch (s.charAt(i)) {
case 'I':
answer = answer + 1;
break;
case 'V':
answer = answer + 5;
break;
case 'X':
answer = answer + 10;
break;
case 'L':
answer = answer + 50;
break;
case 'C':
answer = answer + 100;
break;
case 'D':
answer = answer + 500;
break;
case 'M':
answer = answer + 1000;
break;
}
break;
} else {
switch (s.charAt(i)) {
case 'I':
if (s.charAt(i + 1) == 'V') {
answer = answer + 4;
i++;
} else if (s.charAt(i + 1) == 'X') {
answer = answer + 9;
i++;
} else {
answer = answer + 1;
}
break;
case 'V':
answer = answer + 5;
break;
case 'X':
if (s.charAt(i + 1) == 'L') {
answer = answer + 40;
i++;
} else if (s.charAt(i + 1) == 'C') {
answer = answer + 90;
i++;
} else {
answer = answer + 10;
}
break;
case 'L':
answer = answer + 50;
break;
case 'C':
if (s.charAt(i + 1) == 'D') {
answer = answer + 400;
i++;
} else if (s.charAt(i + 1) == 'M') {
answer = answer + 900;
i++;
} else {
answer = answer + 100;
}
break;
case 'D':
answer = answer + 500;
break;
case 'M':
answer = answer + 1000;
break;
}
}
}
return answer;
}
}
다른 사람의 코드를 살펴보자. 해시맵을 사용하여 짧고 간단하게 푼 풀이 법이다.
각각의 로마자가 가지는 값을 맵에 넣고 rea에 먼저 0번째 값을 더했다. 그리고 index 를 1부터 시작하며 현재 로마자인 curr 과 이전 로마자인 pre를 비교하여 내가 고민했던 index 에러를 해결했다....
public class Solution {
public int romanToInt(String s) {
int res = 0;
HashMap<Character,Integer> map = new HashMap<Character,Integer>();
map.put('I',1);
map.put('V',5);
map.put('X',10);
map.put('L',50);
map.put('C',100);
map.put('D',500);
map.put('M',1000);
res += map.get(s.charAt(0));
for(int i=1;i<s.length();i++){
int cur = map.get(s.charAt(i));
int pre = map.get(s.charAt(i-1));
if (pre<cur) res -= pre*2;
res += cur;
}
return res;
}
}
'Algorithm > LeetCode' 카테고리의 다른 글
LeetCode - 169. Majority Element (0) | 2020.04.04 |
---|---|
LeetCode - 155.Min Stack (JVM 의 메모리 Saving 이슈) (0) | 2020.04.03 |
LeetCode - 141.Linked List Cycle (1) | 2020.04.01 |
LeetCode - 21.Merge Two Sorted Lists (0) | 2020.04.01 |
LeetCode - 20.Valid Parentheses (0) | 2020.03.28 |
댓글